🧑💻 EVM Compatible Blockchain
Ethereum, Polygon, BNB Smart Chain, Klaytn, BORA, MEVerse
All blockchain assets can be transferred using face.Wallet().SendTransaction(rawTransaction)
. The types of assets they want to send, such as Native Coin, ERC20 tokens, and ERC721 are divided by a parameter rawTransaction
. The schema of 'rawTransaction' can be found in here.
The request for transaction responds to the TransactionRequestId
object for its own unique requestId
and transactionId
(Transaction Hash). Detailed schemas can be found in here.
Send Coin
You can use 'Send Coin' feature in the 'Platform Coin Transaction' section on the Sample Dapp. After typing in the amount of coin and receiver address then press the button 'Send Platform Coin', you can see the screenshot as below.
After sending coin the transaction hash is displayed RESULT:
data plain (the 3rd screenshot).
To send native coins (eg Ethereum, Klayton, etc.), you must make a request
as follows. data
must be empty(null
) and value
must be hexadecimal format.
RawTransaction rawTransaction = new RawTransaction(
loggedInAddress,
receiverAddress,
string.Format($"0x{value}"),
null
);
TransactionRequestId transactionRequestId = await face.Wallet().SendTransaction(rawTransaction);
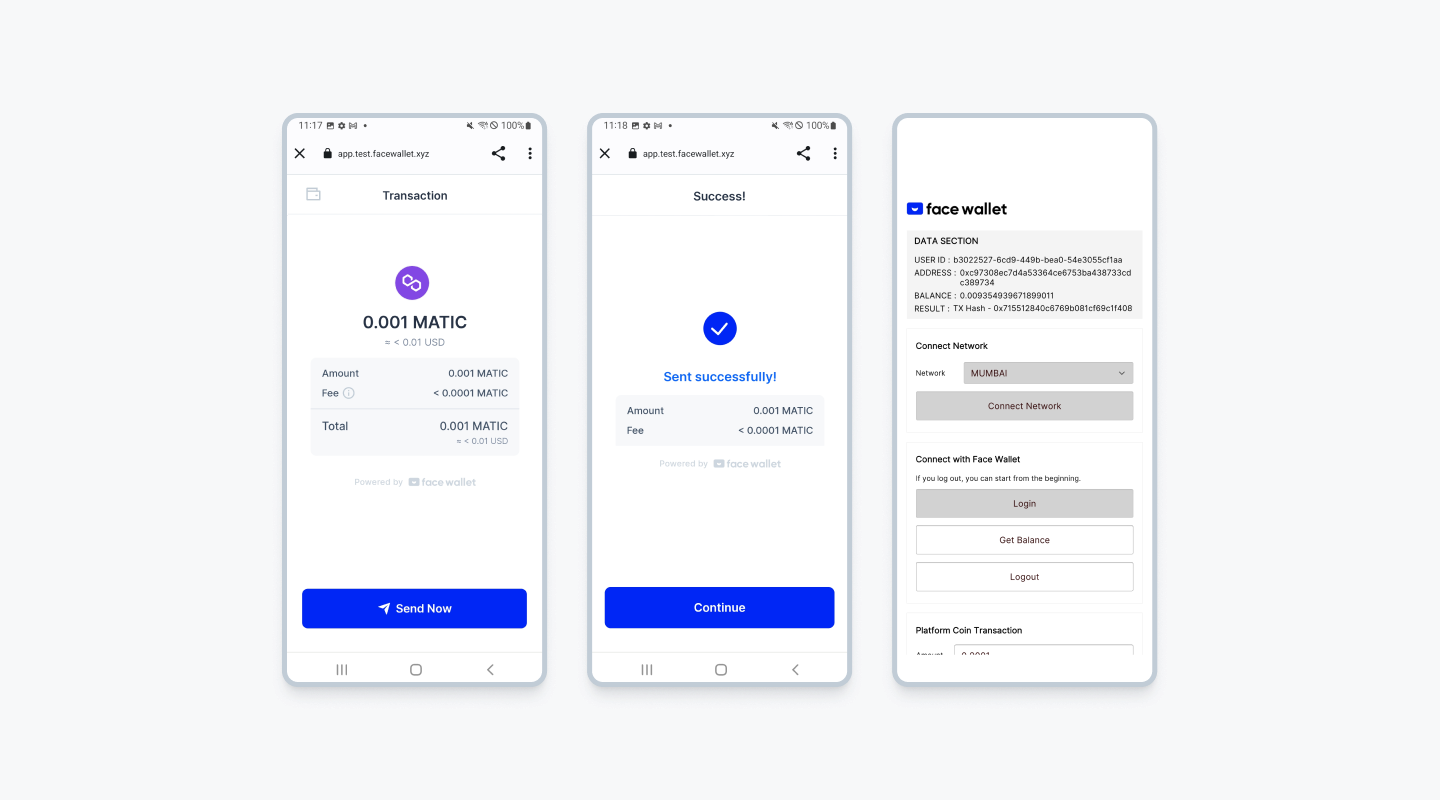
Get Coin Balance
You can find your wallet balance by clicking 'Get Balance' button on the 'Connect with Face Wallet' section. The balance is displayed in RESULT:
data plain.
You can use face.Wallet().GetBalance()
to check the balance of the wallet address.
FaceRpcResponse response = await this.face.Wallet().GetBalance(address);
return NumberFormatter.DivideHexWithDecimals(response.CastResult<string>(), 18);
Send ERC20
To send ERC20 with the Sample Dapp, you can use 'ERC20 Transaction' section.
For ERC20 tokens, you can build the data using face.datafactory.CreateErc20SendData()
.
string data = face.dataFactory.CreateErc20SendData(tokenAddress, receiverAddress, amount, decimals);
RawTransaction rawTransaction = new RawTransaction(
loggedInAddress,
tokenAddress,
"0x0",
data
);
TransactionRequestId transactionRequestId = await face.Wallet().SendTransaction(rawTransaction);
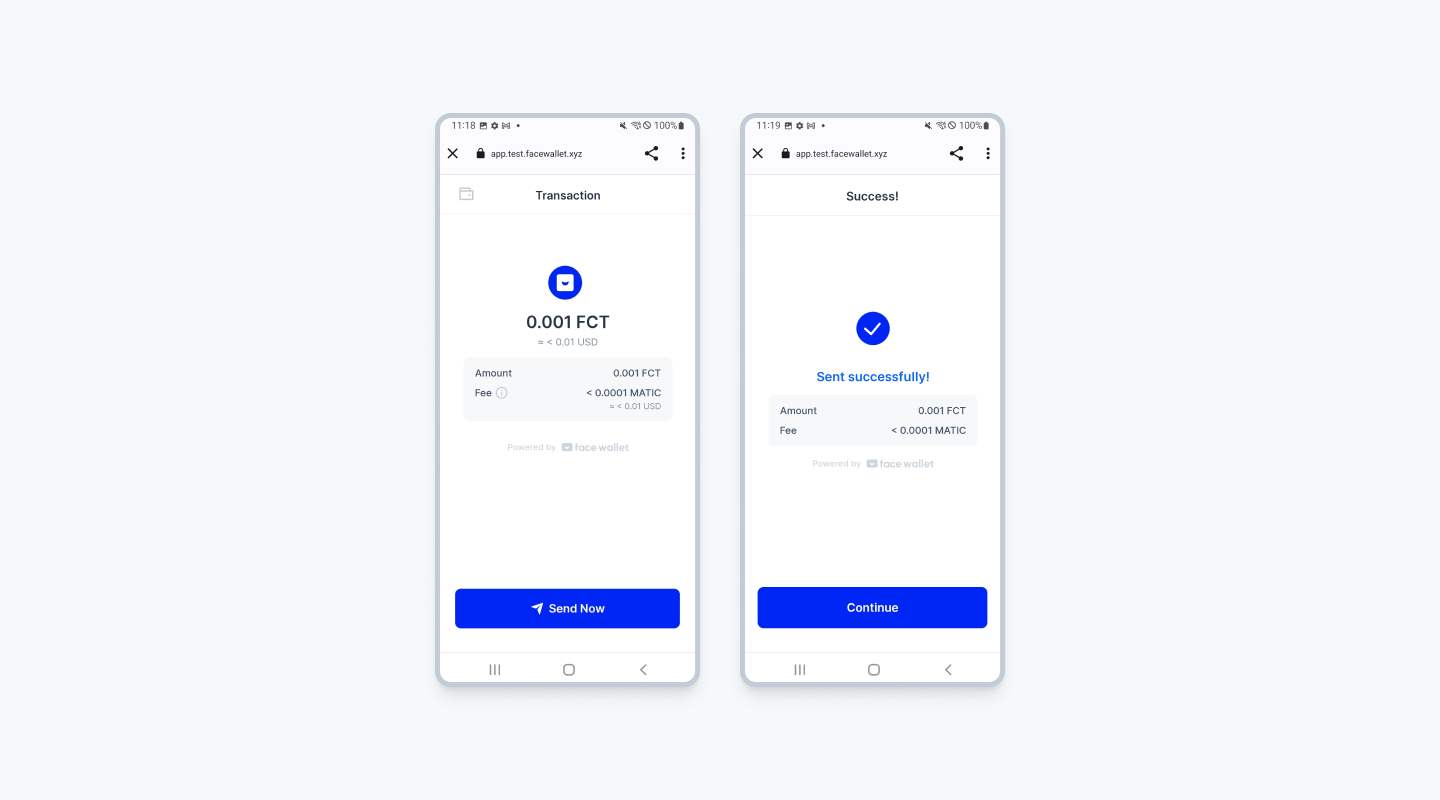
Send NFT
For ERC721 NFT tokens, you can build the data using face.dataFactory.CreateErc721SendData()
string data = face.dataFactory.CreateErc721SendData(nftAddress, loggedInAddress, receiverAddress, tokenId);
RawTransaction rawTransaction = new RawTransaction(
loggedInAddress,
nftAddress,
"0x0",
data
);
TransactionRequestId transactionRequestId = await face.Wallet().SendTransaction(rawTransaction);
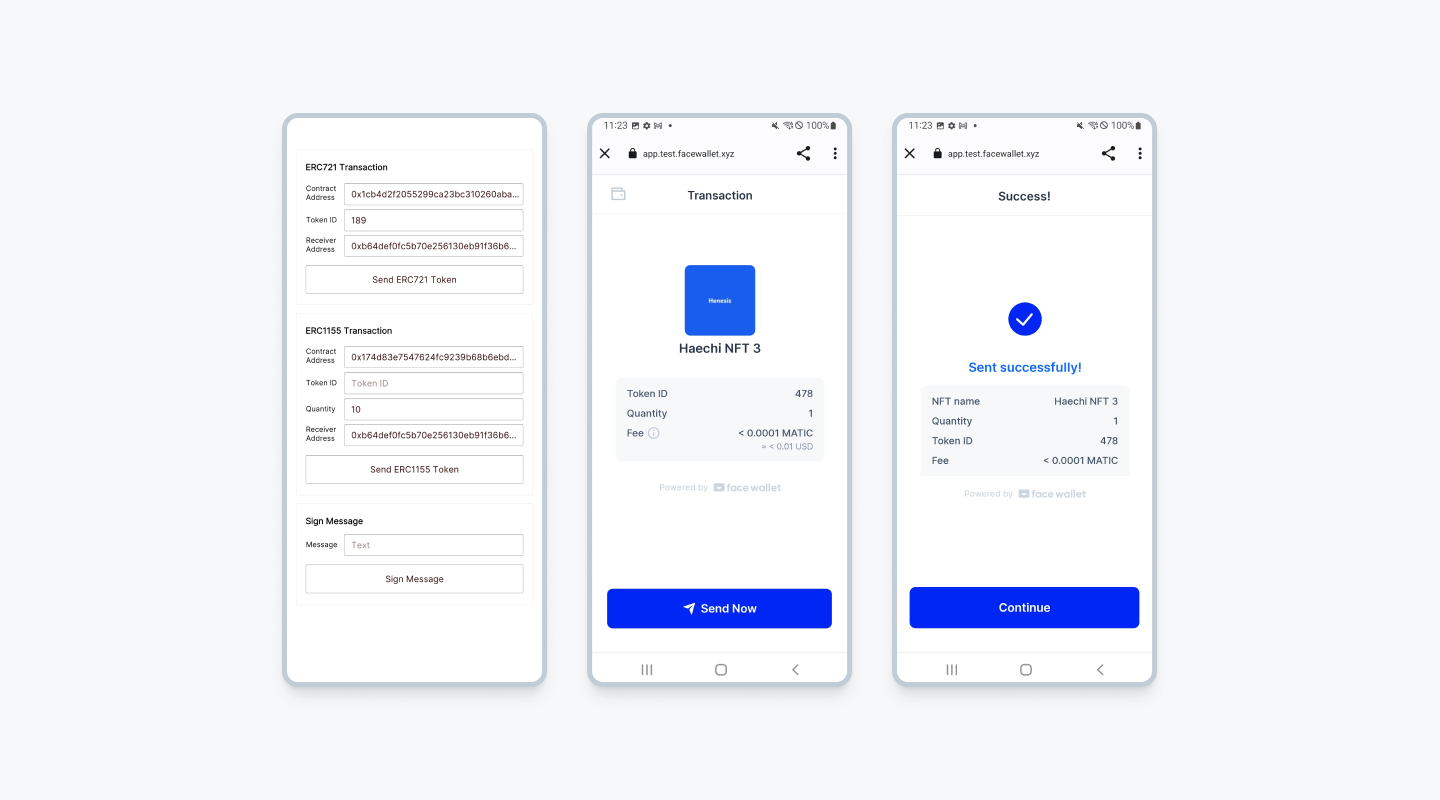
Send ERC1155
For ERC1155 NFT tokens, you can build the data using face.datafactory.CreateErc1155SendBatchData()
.
string data = face.dataFactory.CreateErc1155SendBatchData(
nftAddress,
loggedInAddress,
receiverAddress,
tokenId,
quantity
);
RawTransaction rawTransaction = new RawTransaction(
loggedInAddress,
nftAddress,
"0x0",
data
);
TransactionRequestId transactionRequestId = await face.Wallet().SendTransaction(rawTransaction);
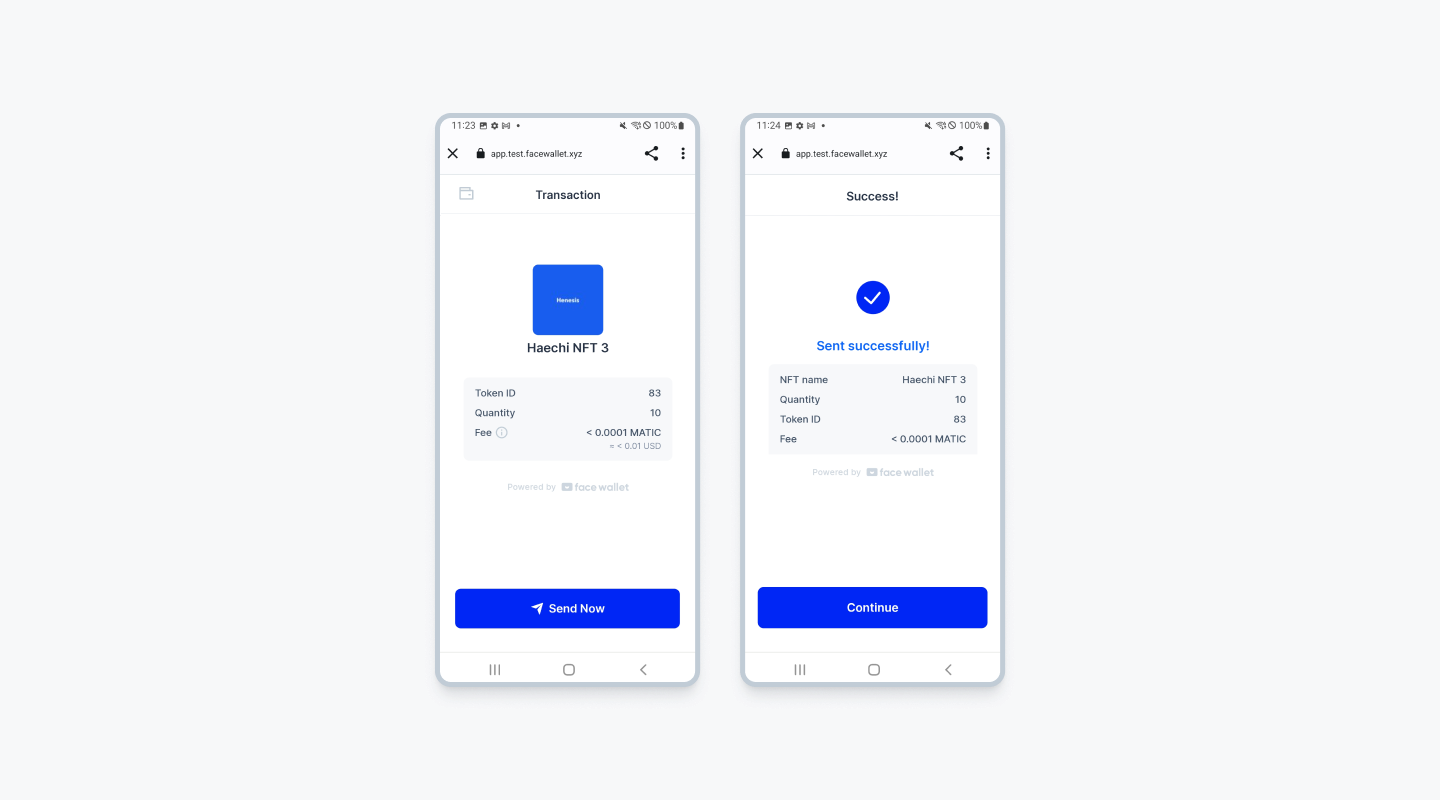
You can use the SDK to sign a message or call the function of the contract you want.
The response to the request is returned in FaceRpcResponse
. You can check the schema of the object in here.
Sign Message
You can sign message to generate a signature.
FaceRpcResponse response = await this.face.Wallet().SignMessage(message);
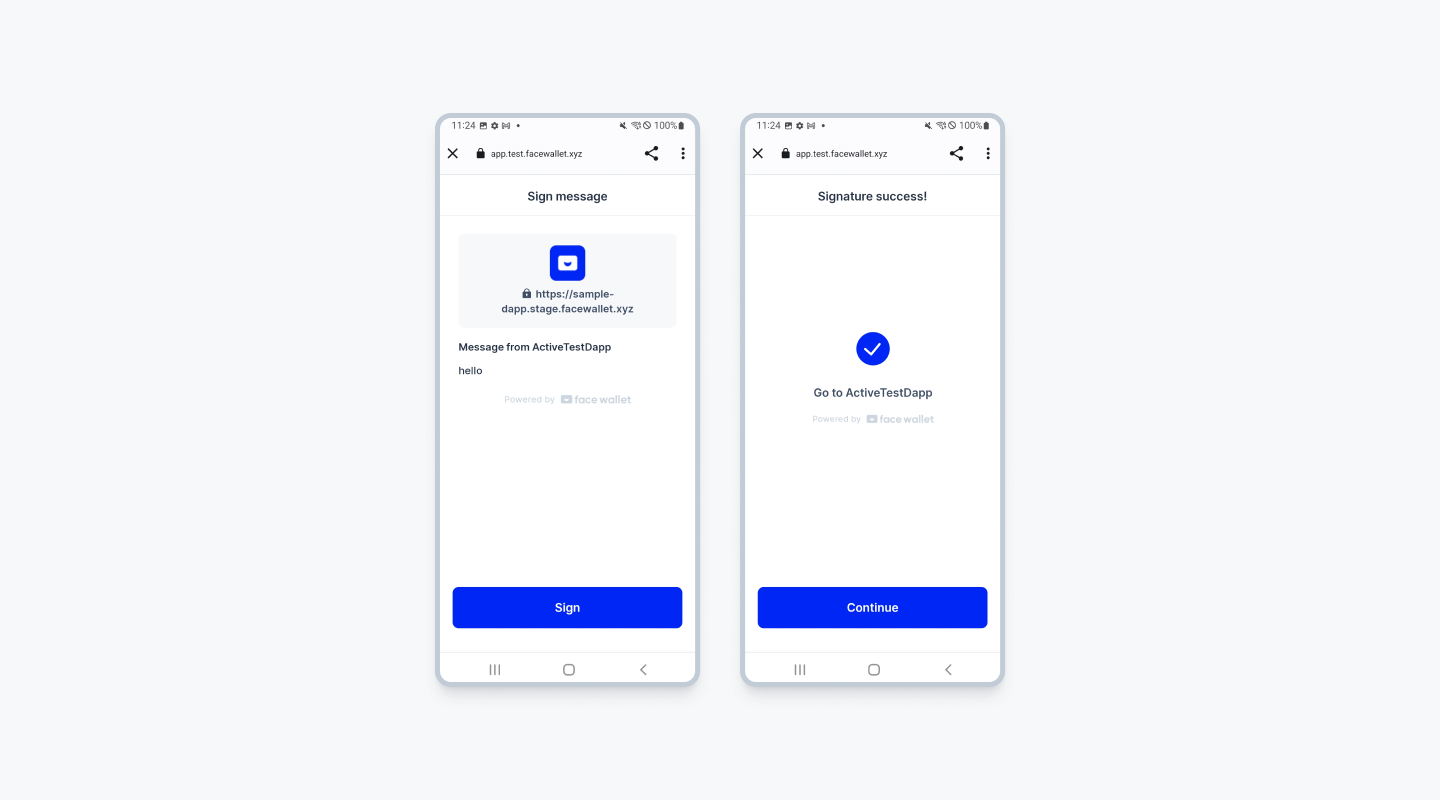
Contract Call
You can initiate a contract call by constructing the desired data. Use face.Wallet().Call(rawTransaction)
to make the request. Below is an example of a contract call to query the balance of an ERC20 token.
string data = face.dataFactory.CreateErc20GetBalanceData(tokenAddress, loggedInAddress);
RawTransaction rawTransaction = new RawTransaction(
null, // from
tokenAddress, // to
"0x0",
data
);
FaceRpcResponse response = face.Wallet().Call(rawTransaction);
You can also interact with other smart contracts by using your own specific ABIs.
For example, here's how to call the custom function of a TestERC1155 contract. Please note that this SDK does not include the contracts being tested.
Nethereum.Contracts.Contract erc1155 = this._web3.Eth.GetContract(TEST_ERC1155_ABI, tokenAddress);
Function mintAutoFunction = erc1155.GetFunction("mintAuto");
string data = mintAutoFunction.GetData(
receiverAddress,
amount,
ABIType.CreateABIType("bytes32").Encode(""));
RawTransaction rawTransaction = new RawTransaction(
fromAddress, // from
tokenAddress, // to
"0x0",
data
);
FaceRpcResponse response = face.Wallet().Call(rawTransaction);
Updated about 1 year ago