🧑💻 Connect Wallet
Initialize
You can use the face.Initialize()
function to set the desired network and environment. Detail API reference can be found in here.
face.Initialize(
new FaceSettings.Parameters
{
ApiKey = "API_KEY",
Network = BlockchainNetwork.ETHEREUM, // or MUMBAI, BNB_SMART_CHAIN, KLAYTN
Scheme = "CUSTOM_SCHEME"
}
);
This work must be executed before executing all features including login. When 'face.initialize' is completed, the following features can be used.
On the Sample Dapp by selecting 'Network' field, it calls face.Initialize()
internally.
Deeplink Scheme Setup (Android)
For using Face Wallet on android, register a deeplink scheme at AndroidMenifest.xml
.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools">
<application>
......
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="CUSTOM_SCHEME" android:host="CUSTOM_SCHEME" />
</intent-filter>
</activity>
</application>
</manifest>
Then, provides the deeplink scheme to face.Initialize()
as a parameter.
Deeplink Scheme Setup (iOS, WebGL)
Provides a deeplink scheme to face.Initialize()
as a parameter.
SignUp/Login
On Sample Dapp, after selecting blockchain network, and click 'Connect Network' button, now we can move on to sign up, login flow.
Currently, Unity SDK provides three ways of SignUp/Login:
Connect Wallet with Id Token
You can use face.Auth().LoginWithIdToken()
for SignUp/Login with a Social Id Token. (The Id Token must be issued from Google.)
When you use LoginWithIdToken()
, you needs a signature signed with API Secret.
string idToken = "id_token"; // issued from google or face supported providers
string sig = "sig"; // signed idToken by API Secret
await face.Auth().LoginWithIdToken(idToken, sig);
For details for creating a signature, please visit here.
How can I get my API Secret?
Please contact and request API Secret to Face Wallet Team.
API Secret should be careful not to leak outside. Also, a signature should be created in your backend, not frontend.
Connect Wallet with Social Login
If you want to SignUp/Login via direct social login, you can use face.Auth().DirectSocialLogin(provider)
.
provider
type can be string
or LoginProviderType
.
FaceLoginResponse response = await face.Auth().DirectSocialLogin(LoginProviderType.Google);
string address = response.wallet.Address;
Connect Wallet with Face Wallet modal
To sign up or log in using the Face Wallet modal, invoke the method face.Auth().Login()
.
You can pass List<LoginProviderType>
to choose specific providers. If this parameter is set to null, all available providers will be displayed in the list.
List<LoginProviderType> providers = new List<LoginProviderType>() {
LoginProviderType.Google,
LoginProviderType.Apple,
LoginProviderType.Facebook };
FaceLoginResponse response = await face.Auth().Login(providers);
string address = response.wallet.Address;
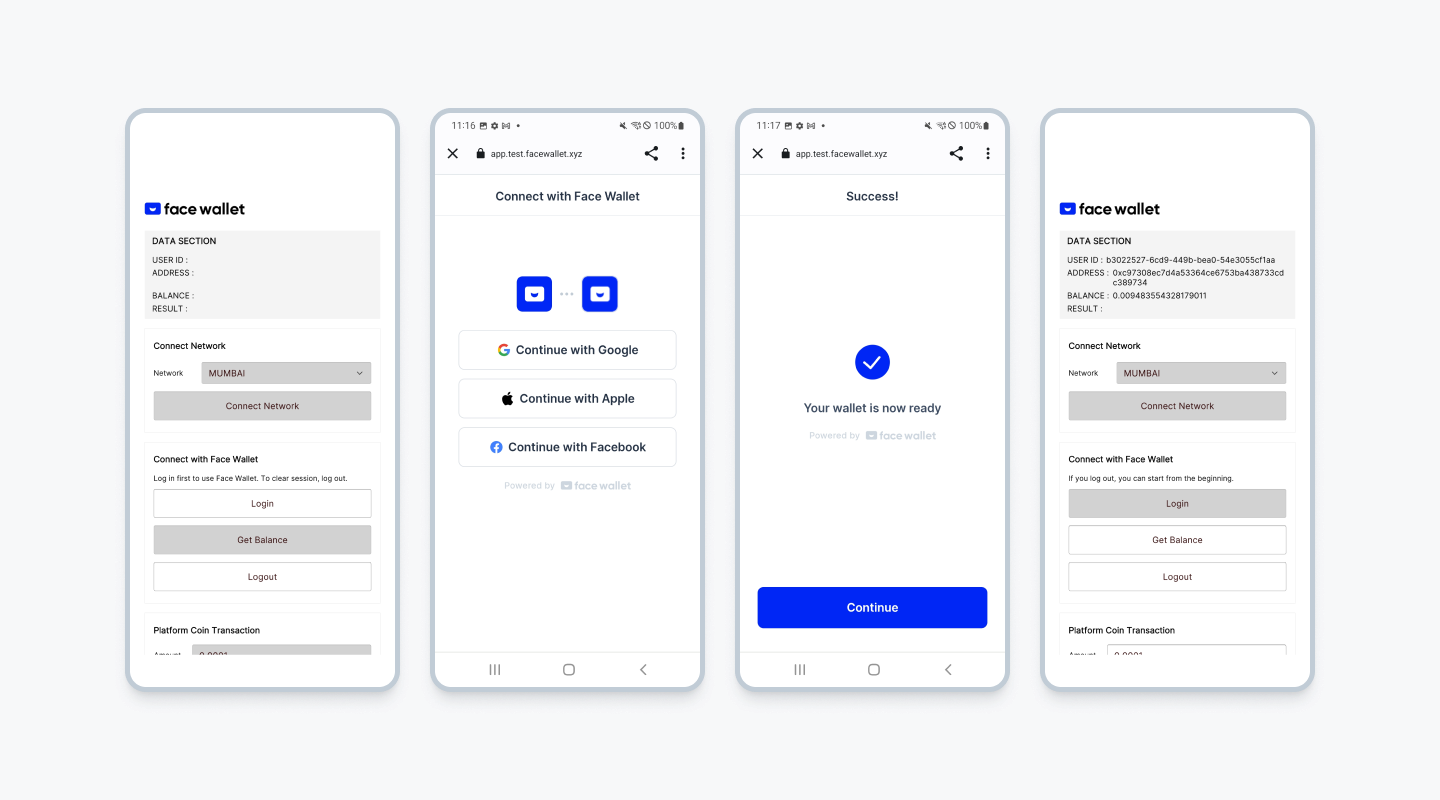
The function responds to the user ID and the user's wallet information in the FaceloginResponse
object.
For more information about FaceLoginResponse
, check here.
Logout
You need to log out to log in to another account when you already logged in. You can log out using face.Auth().Logout()
.
await face.Auth().Logout();
IsLoggedIn
You can check if you are logged in or not, using face.Auth().IsLoggedIn()
. It will return boolean value, true is logged in.
await face.Auth().IsLoggedIn();
Updated about 1 year ago