Smart Contract
By using the actual ABI and Contract object, you can interact with a smart contract. If a contract object is created using the ABI, you can call the smart contract method according to the ABI.
Get data from the smart contract
If the method of the smart contract to be called is read-only (View Function), you can retrieve data by calling the method without a separate transaction.
Below is the code to retrieve data by calling the balanceOf method of the ERC20 smart contract.
import { Face } from '@haechi-labs/face-react-native-sdk';
import { ethers } from 'ethers';
const face = new Face({
network: Network.GOERLI,
apiKey: 'YOUR_DAPP_API_KEY',
scheme: 'CUSTOM_SCHEME'
});
const provider = new ethers.providers.Web3Provider(face.getEthLikeProvider());
const signer = provider.getSigner();
const userAddress = await signer.getAddress();
// ERC20 smart contract
const contractABI = '[{"constant":true,"inputs":[],"name":"name","outputs":[{"name":"","type":"string"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":false,"inputs":[{"name":"_spender","type":"address"},{"name":"_value","type":"uint256"}],"name":"approve","outputs":[{"name":"","type":"bool"}],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[],"name":"totalSupply","outputs":[{"name":"","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":false,"inputs":[{"name":"_from","type":"address"},{"name":"_to","type":"address"},{"name":"_value","type":"uint256"}],"name":"transferFrom","outputs":[{"name":"","type":"bool"}],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[],"name":"decimals","outputs":[{"name":"","type":"uint8"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":true,"inputs":[{"name":"_owner","type":"address"}],"name":"balanceOf","outputs":[{"name":"balance","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":true,"inputs":[],"name":"symbol","outputs":[{"name":"","type":"string"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":false,"inputs":[{"name":"_to","type":"address"},{"name":"_value","type":"uint256"}],"name":"transfer","outputs":[{"name":"","type":"bool"}],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[{"name":"_owner","type":"address"},{"name":"_spender","type":"address"}],"name":"allowance","outputs":[{"name":"","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"payable":true,"stateMutability":"payable","type":"fallback"},{"anonymous":false,"inputs":[{"indexed":true,"name":"owner","type":"address"},{"indexed":true,"name":"spender","type":"address"},{"indexed":false,"name":"value","type":"uint256"}],"name":"Approval","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"name":"from","type":"address"},{"indexed":true,"name":"to","type":"address"},{"indexed":false,"name":"value","type":"uint256"}],"name":"Transfer","type":"event"}]';
const contractAddress = '0x55d398326f99059ff775485246999027b3197955';
const contract = new ethers.Contract(contractAddress, contractABI, signer);
// Get the data of smart contract
const balance = await conatract.balanceOf(userAddress);
Execute a function of smart contract
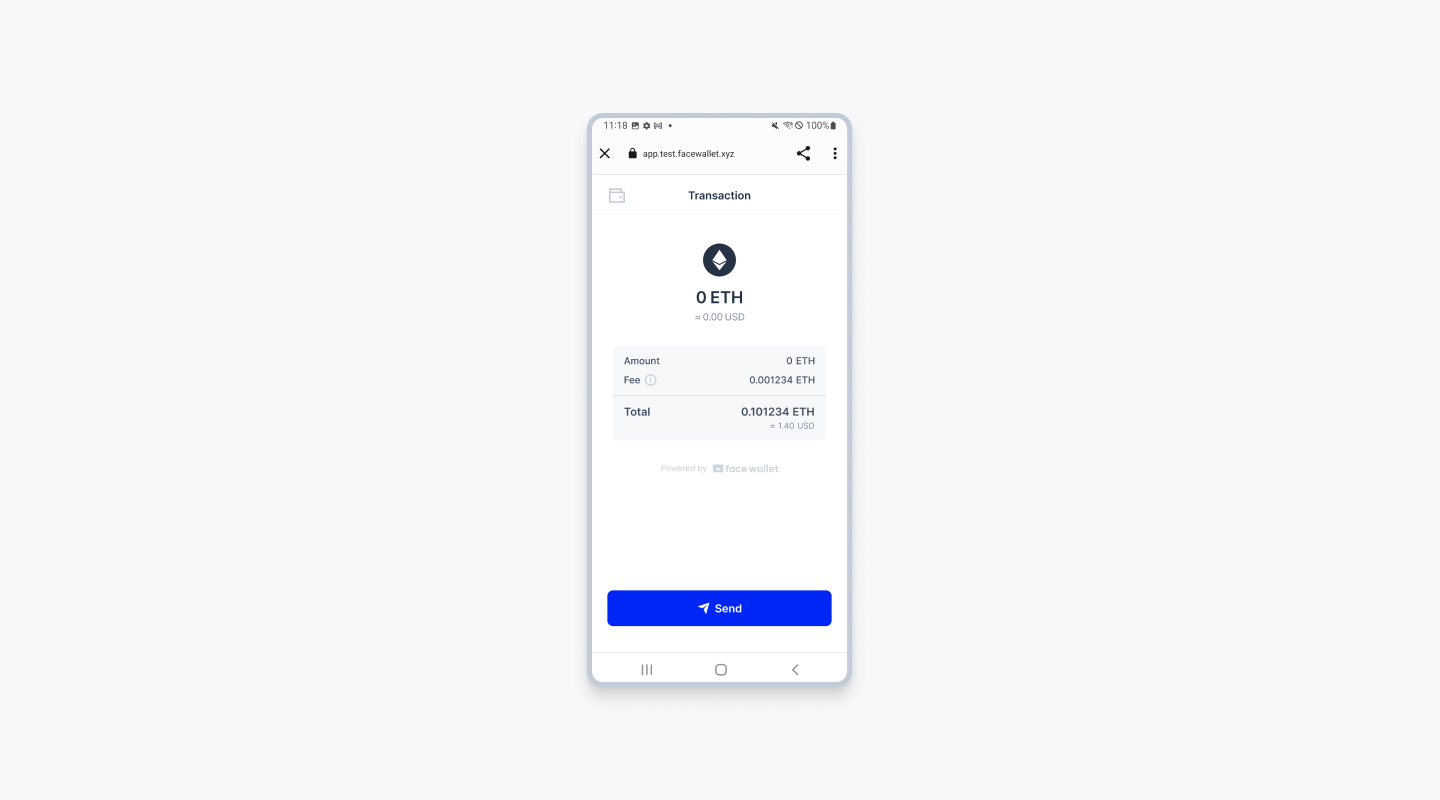
If the method of the smart contract to be called is an operation that requires writing or modifying of blockchain data, a transaction will be sent.
Below is the code to send a transaction by calling the transfer method of the ERC20 smart contract.
import { Face } from '@haechi-labs/face-react-native-sdk';
import { ethers } from 'ethers';
const face = new Face({
network: Network.GOERLI,
apiKey: 'YOUR_DAPP_API_KEY',
scheme: 'CUSTOM_SCHEME'
});
const provider = new ethers.providers.Web3Provider(face.getEthLikeProvider());
const signer = provider.getSigner();
const userAddress = await signer.getAddress();
// Dapp should choose the input required to send transaction
const receiverAddress = '0x9C4206e78bFfca62a4821A5079A7bF46986f6da6';
const amount = ethers.utils.parseUnits('0.1', 18);
// ERC20 smart contract
const contractABI = '[{"constant":true,"inputs":[],"name":"name","outputs":[{"name":"","type":"string"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":false,"inputs":[{"name":"_spender","type":"address"},{"name":"_value","type":"uint256"}],"name":"approve","outputs":[{"name":"","type":"bool"}],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[],"name":"totalSupply","outputs":[{"name":"","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":false,"inputs":[{"name":"_from","type":"address"},{"name":"_to","type":"address"},{"name":"_value","type":"uint256"}],"name":"transferFrom","outputs":[{"name":"","type":"bool"}],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[],"name":"decimals","outputs":[{"name":"","type":"uint8"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":true,"inputs":[{"name":"_owner","type":"address"}],"name":"balanceOf","outputs":[{"name":"balance","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":true,"inputs":[],"name":"symbol","outputs":[{"name":"","type":"string"}],"payable":false,"stateMutability":"view","type":"function"},{"constant":false,"inputs":[{"name":"_to","type":"address"},{"name":"_value","type":"uint256"}],"name":"transfer","outputs":[{"name":"","type":"bool"}],"payable":false,"stateMutability":"nonpayable","type":"function"},{"constant":true,"inputs":[{"name":"_owner","type":"address"},{"name":"_spender","type":"address"}],"name":"allowance","outputs":[{"name":"","type":"uint256"}],"payable":false,"stateMutability":"view","type":"function"},{"payable":true,"stateMutability":"payable","type":"fallback"},{"anonymous":false,"inputs":[{"indexed":true,"name":"owner","type":"address"},{"indexed":true,"name":"spender","type":"address"},{"indexed":false,"name":"value","type":"uint256"}],"name":"Approval","type":"event"},{"anonymous":false,"inputs":[{"indexed":true,"name":"from","type":"address"},{"indexed":true,"name":"to","type":"address"},{"indexed":false,"name":"value","type":"uint256"}],"name":"Transfer","type":"event"}]';
const contractAddress = '0x55d398326f99059ff775485246999027b3197955';
const contract = new ethers.Contract(contractAddress, contractABI, signer);
// Send a transaction to transfer ERC20 token
const balance = await conatract.transfer(userAddress, amount);
Updated 12 months ago