Send Transaction
sendTransaction()
sendTransaction()
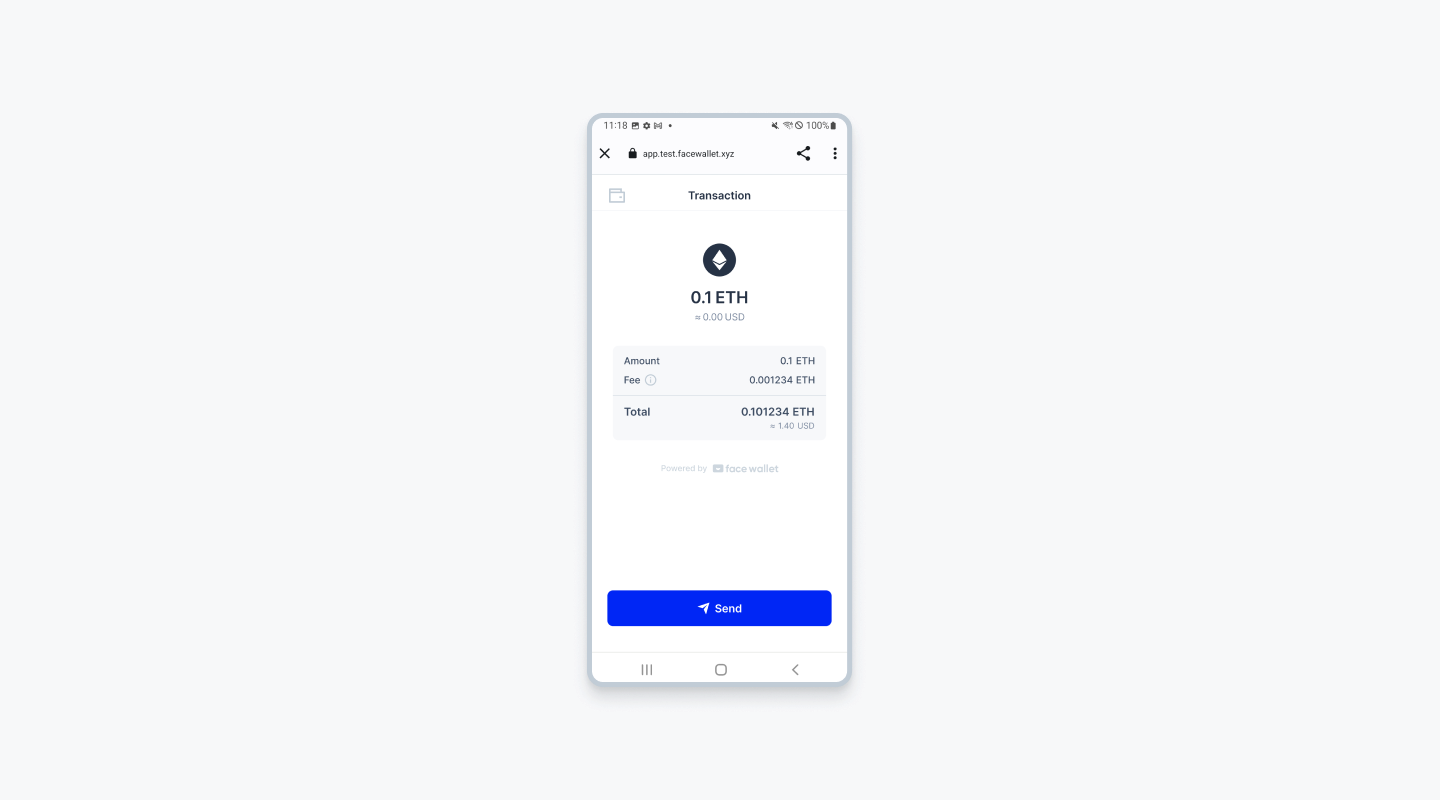
Face wallet webivew to confirm the transaction information
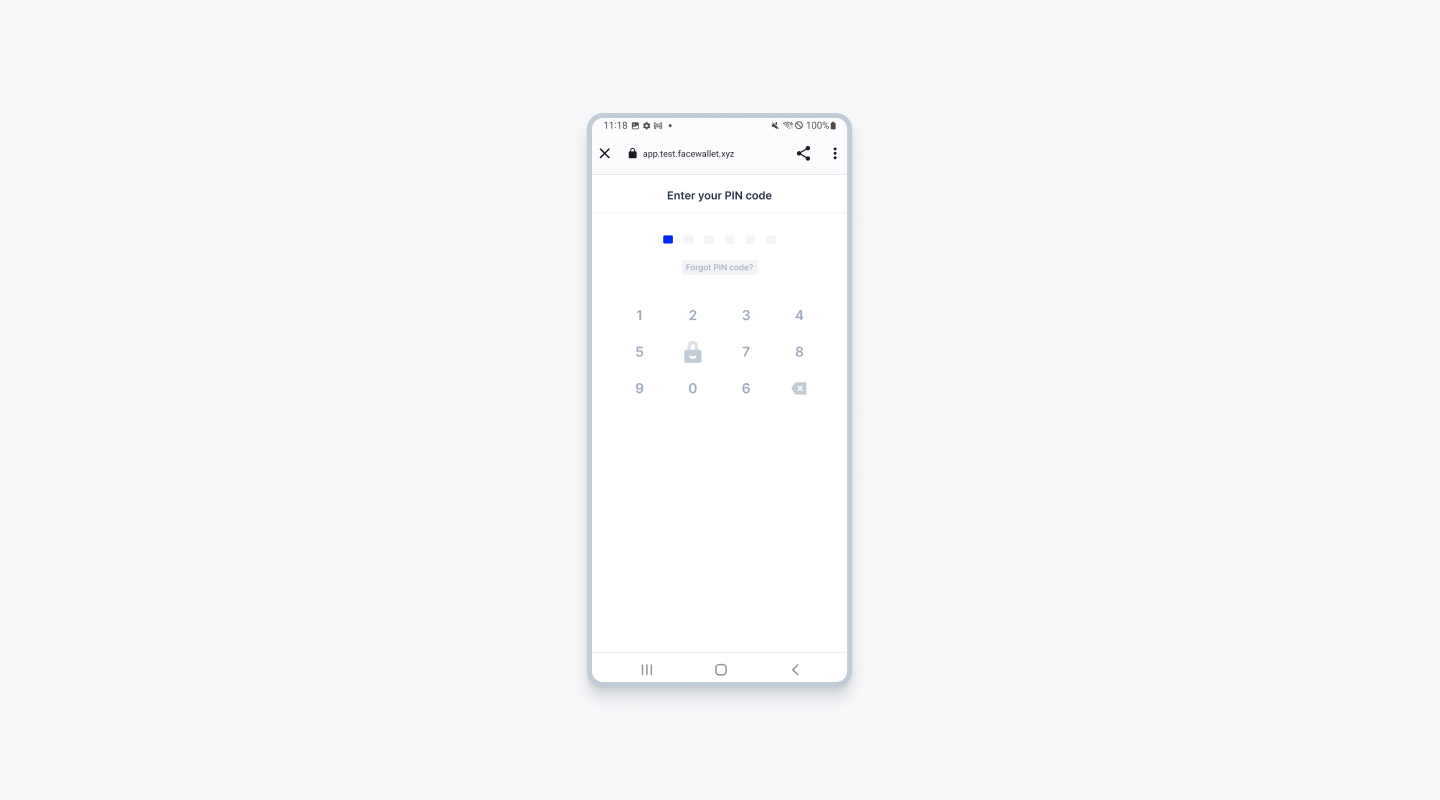
Face wallet webview to get the PIN code from user
If you are currently logged in to the Face Wallet, you can send a transaction from the wallet using Ethers.js. When sending a transaction, the user confirms the transaction information through the Face Wallet webview and enters the PIN code.
If the user is not logged in to the Face Wallet when the sendTransaction()
function of Ethers.js is called, the user will proceed to log in through the Face Wallet webview.
import { Face } from '@haechi-labs/face-react-native-sdk';
import { ethers } from 'ethers';
const face = new Face({
network: Network.GOERLI,
apiKey: 'YOUR_DAPP_API_KEY',
scheme: 'CUSTOM_SCHEME'
});
const provider = new ethers.providers.Web3Provider(face.getEthLikeProvider());
const signer = provider.getSigner();
// Dapp should choose the input required to send transaction
const receiverAddress = '0x9C4206e78bFfca62a4821A5079A7bF46986f6da6';
const amount = ethers.utils.parseEther('0.1');
// This transaction means user send his/her 0.1 ETH to receiver address
// User would confirm the transaction information in Face wallet modal
// Then user would enter the PIN code in Face wallet modal
const tx = await signer.sendTransaction({
to: receiverAddress,
value: amount,
});
// If you want to use 12 confirmations, set 'confirms' parameter to 12
const receipt = await tx.wait();
const txHash = receipt.transactionHash;
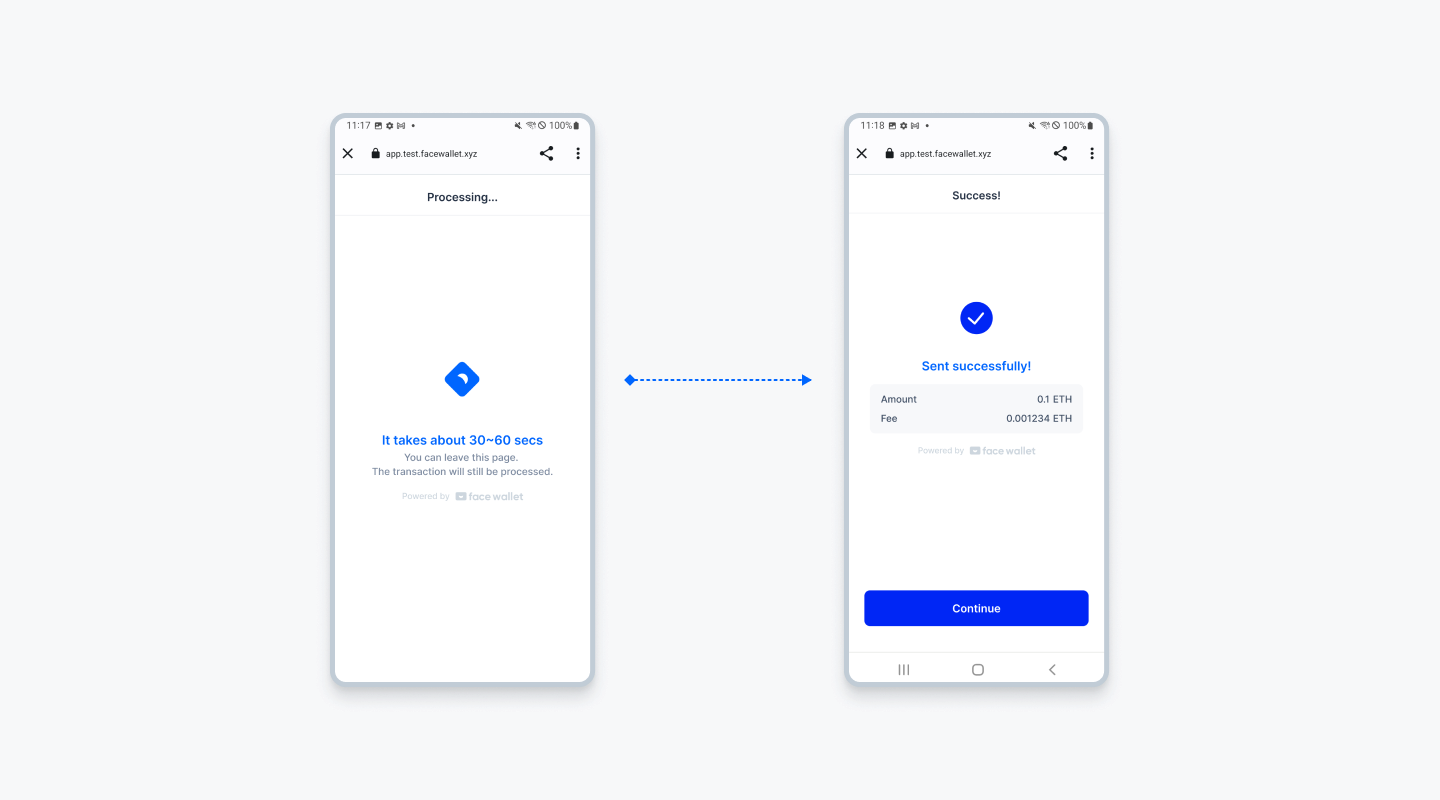
[Processing], [Success] status
When the transaction is sent, the Face Wallet webview switches to the [Processing] status screen. When the user closes the [Processing] screen, Dapp may wait until the transaction is mined through the wait()
method. If the transaction is mined before the user closes the [Processing] screen, the Face Wallet webview switches to the [Success] status screen.
Updated 8 months ago