💡 How to use Hedera Token Service?
1. Register Hedera Token Service Address to Dashboard
To use the "Hedera Token Service," you first need to register the token in the dashboard. If it's a Fungible Token, you can proceed with registration under the "Tokens (ERC20)" tab. For Non-Fungible Tokens, you can register them under the "NFTs (ERC721)" tab.
Mainnet Dashboard URL: https://dashboard.facewallet.xyz/
Testnet Dashboard URL: https://testnet.dashboard.facewallet.xyz/
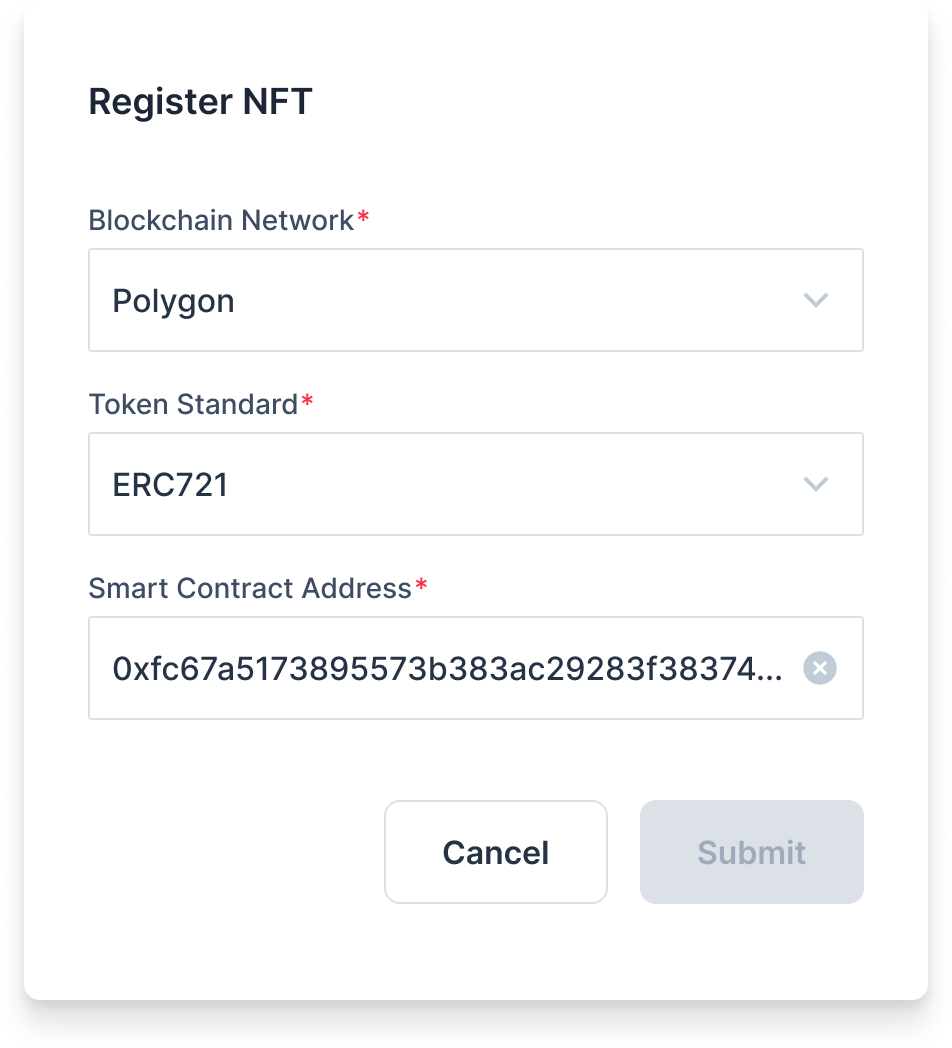
You should enter the Hedera Token Service's EVM address format in the Smart Contract Address field.
Example 0.0.5750380 -> 0x000000000000000000000000000000000057be6c
2. Associate Hedera Token Service
To associate a user's wallet with the Token, please refer to the following link for the process of association.
3. Transfer With Hedera Token Service
You send transactions like transfers to the Hedera Token Service's contract.
import { Face, Network } from '@haechi-labs/face-sdk';
import { ethers } from 'ethers';
const face = new Face({
network: Network.HEDERA,
apiKey: 'YOUR_DAPP_API_KEY'
});
const provider = new ethers.providers.Web3Provider(face.getEthLikeProvider());
const signer = provider.getSigner();
const userAddress = await signer.getAddress();
// Dapp should choose the input required to send transaction
const receiverAddress = '0x9C4206e78bFfca62a4821A5079A7bF46986f6da6';
const amount = ethers.utils.parseUnits('0.1', 18);
// Hedera Token Service Contract
const contractAddress = '0x55d398326f99059ff775485246999027b3197955';
const contract = new ethers.Contract(contractAddress, HEDERA_TOKEN_ABI | HEDERA_NFT_ABI, signer);
// Send a transaction to transfer hedera token service
const balance = await contract.transfer(userAddress, amount);
This is a sample of the Hedera Token Service ABI. (The actual Hedera Token Service ABI may differ from this sample.)
export const HEDERA_TOKEN_ABI = [
'function transfer(address to, uint256 value) public returns (bool success)',
'function balanceOf(address account) view returns (uint256 balance)',
'function approve(address _spender, uint256 _value) public returns (bool success)',
'function allowance(address _owner, address _spender) public view returns (uint256 remaining)',
];
export const HEDERA_NFT_ABI = [
'function transferFrom(address from, address to, uint256 tokenId)',
'function safeTransferFrom(address from, address to, uint256 tokenId)',
'function safeTransferFrom(address from, address to, uint256 tokenId, bytes data)',
'function mintAuto(address to)',
'event Transfer(address indexed from, address indexed to, uint256 indexed tokenId)',
];
Updated over 1 year ago